Interviews are often unpredictable, and when it comes to programming, it is even trickier. JavaScript interviews can be overwhelming as the subject is vast and you won’t know which questions can deceive you. If you plan well then there’s nothing to be anxious about.
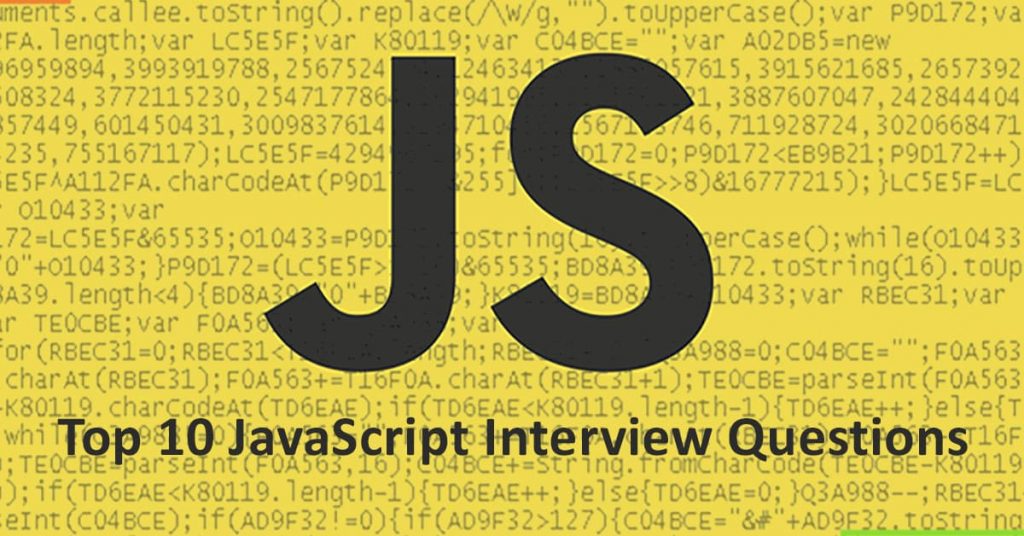
JavaScript Interview Questions Commonly Asked
To help you with that we lay down the questions that are frequently asked. Here are Top 10 JavaScript Interview Questions commonly asked with their answers.
Question No.1: What is the difference between “let” and “var” keyword?
Ans:
This is a fundamental question & an interviewer would expect you to answer it. “var” & “let” are used for the function declaration, but they differ in scope.
- var belongs to function scope whereas let belongs to block scope. “let” terminates/ends wherever “block” scope is defined. Meanwhile, “var” will only end with function scope.
- var has been in JS since beginning whereas let was introduced in ES2015/ES6.
- Variables defined with/using “var” are hoisted at the top of its function. But, variables defined with “let” do not get hoisted.
Question No.2: What is the difference between “undefined” & “null” keywords?
Ans:
Undefined is a keyword without any assigned value. While null is just an assignment value, the programmer can assign a null value to any keyword. Both are two distinct types; typeof(undefined) remains undefined whereas typeof(null) becomes an object.
There’s another dimension to this question as follows:
How is undefined==null true?
Ans:
“==” compares value of both sides. Here, undefined & null both have empty values or no values. Therefore, the comparison is true. Meanwhile, undefined === null is false because “===” (strict equality operator) compares type & value. So, undefined remains undefined but null becomes object.
undefined == null ——> True
undefined === null ——> False
Example:
‘1’ == 1 --—> True
‘1’ === 1 --—> False
Question No.3: State difference between ViewState & SessionState?
Ans:
- ViewState is stored within the page whereas SessionState is in the server.
- ViewState handles data specifically of a webpage & SessionState contains data of a specific session
- SessionState clears itself after an amount of inactivity time. ViewState stores data in hidden fields & has no expiration date.
Question 4: How to access elements using JavaScript?
Ans:
Elements can be retrieved using JavaScript by following functions:
- getElementById()
- getElementByName()
- getElementByValue()
- getElementByTag()
Question 5: What do you mean by Object.freeze & Object.seal?
Ans:
Object Seal:
- Prevents addition and/or removal of properties from seal objects. And, use of delete will return false.
- Existing properties are non-configurable
- Defined properties can be changed
- Seal allows Read & Update but no creation or deletion
Object Freeze:
- Object is immutable
- No changes in defined properties except objects
- Freeze only allows READ
Question 6: What is prototypal inheritance?
Ans:
IMPORTANT
JavaScript objects have the hidden property known as “[[prototype]].”. This prototype refers to an object or its null. Such objects are known as a prototype. To understand prototypal inheritance lets consider an example. Suppose we want to read/view property of an object, but it’s missing. JavaScript by-default calls it from the prototype. Inheritance of properties by default is known as “Prototypal Inheritance.”
Easier Explanation:
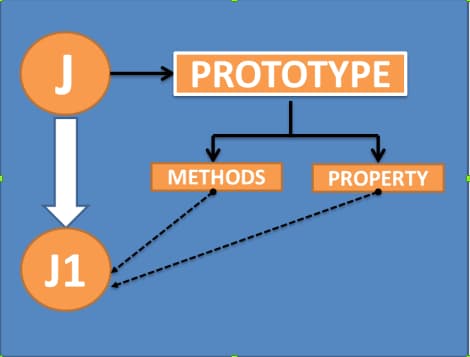
In JavaScript, by default, each function has a particular property known as “Prototype.” By default prototype is empty. However, we can add methods & properties in it. Suppose there’s a function “J.” And its prototype has methods & properties in it. Now, if we create an object using “J” say J1. J1 will inherit properties & methods from “J.” This type of inheritance is known as “Prototypal Inheritance.”
Question 7: What is the difference between function declaration & function expression?
Ans:
Function declaration & function expression are two different entities. The major difference is “Hoisting” & “Syntax”.
- Function declaration syntax : function function_name().
Function expression syntax : anonymous function (like let, var, etc) function name() - Function declaration: declares a function.
Function expression: defines a function & can be stored in a variable. - A function declaration can load before its definition, but function expression will only load when the interpreter reaches the code line.
- Function expression behaves like a variable & has a variable scope. But, the declaration doesn’t possess such things.
- Function expressions can be passed to another function because it behaves like a variable.
Question 8: What is promise & why do we use it?
Ans:
As the name says, PROMISE represents a value that is not present but will be available in the future. A promise represents asynchronous code operations. Programmers use Promise as an alternative of composing, executing or handling asynchronous operation instead of call-back operations. It is easier to handle such operations using Promise because it provides approaches similar to synchronous catch/try.
Phases/States of Promise:
Pending: Asynchronous operation not completed
Fulfilled : Operation synchronized & value determined
Rejected: Operation failed!
Question 9: What is Regular Expression (regExp)?
Ans:
- Set of characters sequentially arranged to form a search pattern is known as “Regular Expression.”
- The programmer can use this search pattern to search for data in the text.
- Regular Expressions can have an intricate pattern or a simple single character.
- Can perform a text search & text replace operation of all types.
Question 10: What is the difference between Client-side & Server-side JavaScript?
Ans:
Scripts can be written in 2 sides; Client & Server. The significant difference between server-side & client-side is “back end.” In server-side, there’s involvement of server whereas in client-side it requires browser on the user end.
Client-Side JavaScript:
- Runs script on the system after the webpage is loaded
- Server Interaction is low
- User Interactivity is high
- It does not allow reading or writing of files
- It doesn’t comprise Multi-processor or threaded capabilities
Server-Side JavaScript:
- Runs on the server-side
- Never gets downloaded to the browser
- Availability of languages like JSP, ASP, etc
- Can validate data on both ends; client (for user validation) and server (for security).
Few more important topics you should not miss :
- Map()
- Reduce()
- Filter()
- Event Bubbling
- Hoisting
- Callbacks
- Global, function & object scopes (Basic)
- JavaScript scopes especially Closures
- Bind, apply & call
Conclusion:
These questions are subjective. From an interviewer’s perspective, they might expect you to answer such questions. This compilation covers the top 10 irrespective of its depth or complexity. In the end, there are few key/crucial terms an individual should know for a JS Interview. That’s all! Best of luck!